NetNow provides a UI component to consume Payment Intents.
Setup
yarn add @netnowfinancial/netnow-react-sdk
// OR
npm install @netnowfinancial/netnow-react-sdk
Usage
The package includes NetNowView
. This view includes a modal and a customizable button which opens it. The button is customizable so that you can provide your own ButtonComponent and keep the design more consistent with your theme. Here's an example:
import { NetNowView, PaymentIntent, ClickableProps } from '@netnowfinancial/netnow-react-sdk';
export default Checkout = ({}) => {
const Button = ({ children, onClick }: ClickableProps) => {
return (
<div
style={{
height: "48px",
width: "200px",
border: "solid",
borderWidth: "2px",
borderRadius: "16px",
}}
onClick={onClick}
>
{children}
</div>
);
};
const confirmIntent = (paymentIntent: PaymentIntent) => {
// call backend. see `Confirm Payment Intent`
}
// see `Create Payment Intent`
const paymentIntent = fetchPaymentIntent()
// Here is a mocked intent
// const paymentIntent = {
// id: '123',
// buyer: { id: '321' },
// amount: 527689,
// currency: 'CAD',
// net_terms: 30,
// fee: 250,
// status: 'initial',
// created: new Date(),
// }
return <NetNowView
ButtonComponent={Button}
modalOpenOverride={false} // use this to open/close the modal manually, for example after intent is confirmed
paymentIntent={paymentIntent}
onConfirmPayment={() => confirmIntent(paymentIntent)}
/>;
}
Which will render:
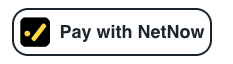
What if I don't want to show this button?
To hide this button, for example in the case that you don't want to offer payment with net terms to a specific buyer or on a specific item, set
paymentIntent
toundefined
in the props.
Clicking on the button will open the following modal:
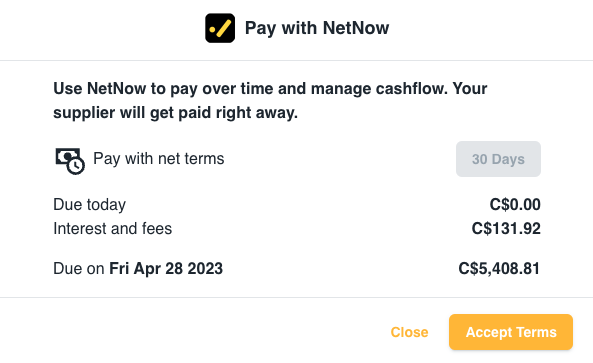